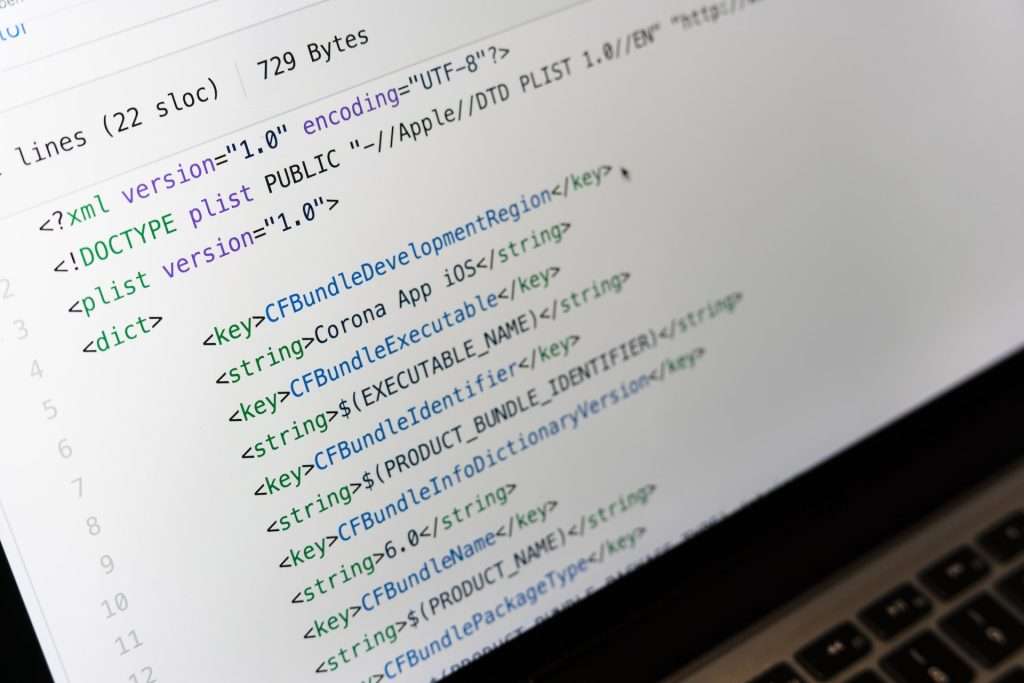
In today’s digital age, having a personal portfolio website is a powerful tool for showcasing your skills, projects, and achievements to the world. In this article, we will walk through the process of creating a simple portfolio website using HTML and CSS, and then we’ll learn how to host it for free on GitHub Pages. This website will have a header section, a Skills section, a Projects section, an Education section, a Contact Me section, and a footer. Let’s get started!
Setting Up the Basic Structure
First, let’s create the basic structure of your portfolio website using HTML. We’ll start with the header section, which will include your name and a profile picture.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css"> <!-- We'll create this file later for CSS styling -->
<title>Your Portfolio</title>
</head>
<body>
<header>
<h1>Your Name</h1>
<img src="your-image.jpg" alt="Your Name">
</header>
<!-- Add other sections here -->
<footer>
© 2023 Your Portfolio
</footer>
</body>
</html>
Replace "your-image.jpg"
with the filename of your profile picture. We’ll cover how to upload this image to GitHub later.
Adding Your Skills
In the Skills section, you can list your skills using an unordered list.
<section>
<h2>Skills</h2>
<ul>
<li>HTML</li>
<li>CSS</li>
<li>JavaScript</li>
<!-- Add more skills as needed -->
</ul>
</section>
Showcasing Your Projects
Next, create an ordered list to display your projects in the Projects section.
<section>
<h2>Projects</h2>
<ol>
<li>Portfolio Website</li>
<li>E-commerce App</li>
<li>Blog Website</li>
<!-- Add your projects here -->
</ol>
</section>
Highlighting Your Education
Use an HTML table to present your education details in a structured way.
<section>
<h2>Education</h2>
<table>
<tr>
<th>Qualification</th>
<th>Institution</th>
<th>Year</th>
</tr>
<tr>
<td>Bachelor's Degree</td>
<td>Your University</td>
<td>20XX</td>
</tr>
<tr>
<td>Master's Degree</td>
<td>Your University</td>
<td>20XX</td>
</tr>
<!-- Add more rows for additional education details -->
</table>
</section>
Contact Me
To allow visitors to get in touch with you, create a Contact Me section with a form.
<section>
<h2>Contact Me</h2>
<form action="https://formspree.io/your-email" method="POST">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="message">Message:</label>
<textarea id="message" name="message" required></textarea>
<input type="submit" value="Submit">
</form>
</section>
Replace "https://formspree.io/your-email"
with the URL provided by Formspree, a service that allows you to receive form submissions via email.
Styling Your Portfolio Website with CSS
To make your portfolio website visually appealing, you’ll need to apply styles using CSS (Cascading Style Sheets). Let’s create a separate CSS file and link it to your HTML document.
Create a CSS file: Create a new file named “style.css” in the same directory as your HTML file.
Link the CSS file to your HTML: In the
<head>
section of your HTML document, add the following line to link the CSS file:
<link rel="stylesheet" href="styles.css">
- Add CSS styles: In the “style.css” file, you can define styles for various elements. Here’s an example of how to style the header section:
/* Add a background color and adjust padding for the header */
header {
background-color: #007BFF;
color: #fff;
text-align: center;
padding: 40px 0;
}
/* Style the header text */
header h1 {
font-size: 36px;
margin-bottom: 10px;
}
/* Style the profile image */
header img {
width: 150px;
height: 150px;
border-radius: 50%;
border: 4px solid #fff;
}
/* Style the navigation menu */
nav ul {
list-style-type: none;
padding: 0;
margin: 20px 0;
}
nav li {
display: inline;
margin-right: 20px;
}
/* Add hover effect to navigation links */
nav a:hover {
text-decoration: underline;
}
/* Style the sections with a subtle background color and padding */
section {
background-color: #f9f9f9;
padding: 30px;
margin-bottom: 30px;
border-radius: 5px;
box-shadow: 0px 2px 5px rgba(0, 0, 0, 0.1);
}
/* Style the section headings */
section h2 {
font-size: 24px;
margin-bottom: 20px;
color: #333;
}
/* Style the project list with icons */
ul.projects {
list-style-type: none;
padding-left: 0;
}
ul.projects li {
margin-bottom: 10px;
position: relative;
}
ul.projects li::before {
content: "\2022"; /* Bullet character */
color: #007BFF; /* Bullet color */
font-size: 16px;
position: absolute;
left: -20px;
top: 6px;
}
/* Style the table */
table {
width: 100%;
border-collapse: collapse;
margin-bottom: 20px;
background-color: #fff;
border: 1px solid #ddd;
border-radius: 5px;
box-shadow: 0px 2px 5px rgba(0, 0, 0, 0.1);
}
/* Style table headers */
th {
background-color: #007BFF;
color: #fff;
font-weight: bold;
padding: 10px;
text-align: left;
}
/* Style table data cells */
td {
padding: 10px;
border: 1px solid #ddd;
}
/* Add zebra striping to table rows for better readability */
tr:nth-child(even) {
background-color: #f2f2f2;
}
/* Style form labels */
form label {
display: block;
margin-bottom: 10px;
font-weight: bold;
color: #333;
}
/* Style text input fields */
form input[type="text"],
form input[type="email"],
form textarea {
width: 100%;
padding: 10px;
margin-bottom: 20px;
border: 1px solid #ccc;
border-radius: 5px;
font-size: 16px;
}
/* Style the submit button */
form input[type="submit"] {
background-color: #007BFF;
color: #fff;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
form input[type="submit"]:hover {
background-color: #0056b3;
}
/* Style the footer */
footer {
background-color: #333;
color: #fff;
padding: 20px 0;
text-align: center;
}
/* Style links in the footer */
footer a {
color: #fff;
text-decoration: none;
margin: 0 10px;
}
footer a:hover {
text-decoration: underline;
}
This CSS code sets the background color, text color, font size, and styles the profile picture within the header section.
- Continue styling: You can apply styles to other sections, lists, tables, and form elements as needed. Use CSS to customize fonts, colors, margins, padding, and more to create a cohesive and visually appealing design for your portfolio.
Remember that CSS offers a wide range of styling options, so you can get creative with how you want your portfolio to look. Don’t forget to preview your website in a web browser to see how the styles are applied and make adjustments as necessary.
By following these steps, you’ll be able to style your portfolio website and make it uniquely yours.
Finally, in the footer, provide copyright information and a link to your GitHub repository where you will host your portfolio website.
To upload your image and host your website on GitHub Pages, follow these steps:
- Create a GitHub account if you don’t have one.
- Create a new GitHub repository and name it something like “your-username.github.io,” where “your-username” is your GitHub username.
- Now, you’ll be on the repository page. Click on the “Add file” button, then select “Upload files.”
- You can either drag and drop your image files into the designated area or click on “choose your files” to browse for the image on your computer.
- Once you’ve selected your image files, scroll down to the “Commit changes” section. You can add a brief description of your commit if you like. Click the “Commit changes” button to upload your images.
- After committing the changes, your image files will be uploaded to your repository.
- To get the URL of your image, click on the image file you want to use. You’ll see your image displayed on a page. Right-click on the image and select “Copy image address” or “Copy image location” (the exact option may vary depending on your web browser). Now, you can use this URL to display the image in your HTML article.
- Commit your HTML and CSS files to the repository.
- Go to the “Settings” tab of your repository.
- Under the “GitHub Pages” section, set the source branch to “main” (or “master”).
- Your website should now be accessible at “https://your-username.github.io.”
That’s it! You’ve created a simple portfolio website using HTML and CSS and hosted it on GitHub Pages. Now, you can customize the website’s styling using CSS and continually update your portfolio to showcase your latest work and skills. Good luck with your portfolio!
Click here to get the complete source code.